Model, View, Controller, also known as MVC, is a software pattern used by developers to separate concerns between components. In a typical scenario you may want to separate the user interface code from the model data. This gives you many advantages such as removing tight dependencies between classes.
Below is an example of the MVC pattern with ASP.NET MVC. In a normal scenario, a web server will just return any static resource, but ASP.NET MVC sits in the middle of the request pipeline. This process uses logic, in the controller, to determine what data, the model, to send to the web page, the view. This gives you allot of control over what data is sent to the user.
Click here to check out all my content on ASP.NET.
The MVC Process
Below is a diagram that shows how this process would flow.
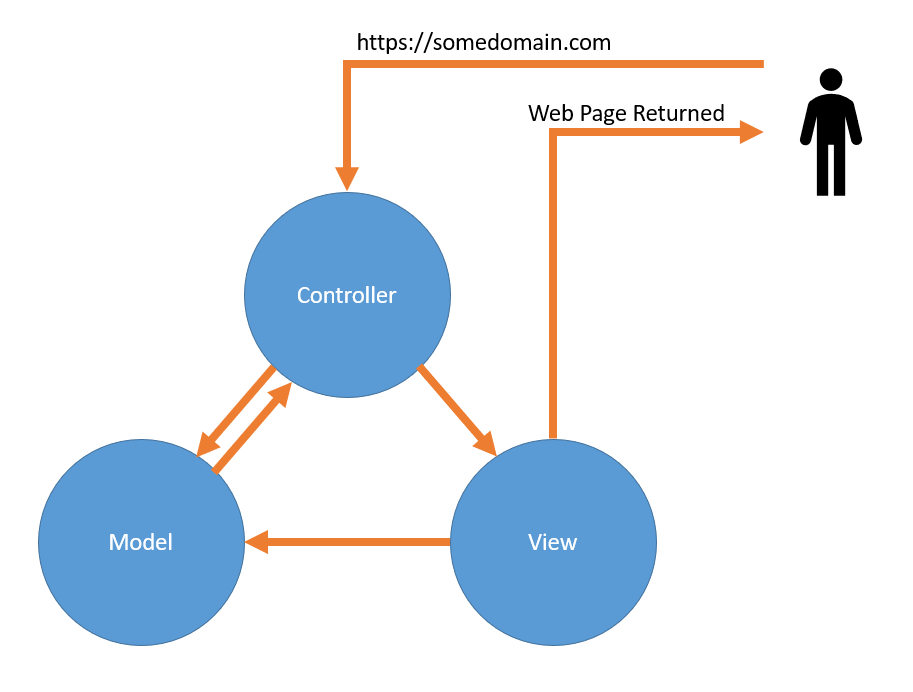
- First, the user will request a web page in the form of a URL
For example https://somedomain.com.
- Second, the Controller will send a request to retrieve the Model data.
This is typically in the form a database request using SQL or maybe even a request to a REST service. That Model data is then returned back to the Controller.
- Third, the Controller will then send the Model data to the View.
The Controller has the opportunity to determine what parts of the data it will send to the View and it also has the opportunity to determine what View it would like to return to the user.
- Finally, the Model data and View will married together and returned to the user.
Views are typically very simple and do not contain any business logic.
Ok, so now we understand the flow of this process, lets dig into each of these major components the MVC pattern.
The Controller
Think of the controller as the brains. Now, this might seem kind of odd, because normally when someone is requesting a webpage, they’re going straight to a webpage, and if there is any server side logic on the page, it will execute this logic before returning the web page. This process creates a tight coupling between the web page and logic.
What if I wanted to change the layout of the web page from some of my user and not of users? This task becomes very difficult as the code and web page are tightly coupled.
With a controller we can write logic to determine what data and what view is returned at execution time.
The Model
This model, or data, is pretty straight forward to understand. Model data is typically requested by the controller, not the view. The goal is to keep the Model and the View as two separate objects so that they remain independent of each other.
Let’s say, for example, we’re going to display a list of products. So our model may reach out to a database and query for a list of those products. Maybe the user is only able to view specific products, so our model will filter the products they are allowed to see.
Sometimes you may also see another pattern used here called the repository pattern, which is often used to separate our the logic of retrieving data.
Once all data is gathered, it is then sent back to the controller.
The View
Once the view receives the model data from the controller it can then display it, typically in the form of HTML. However, you can return other types of data. For example, the view can return data in XML or even JSON format depending on your needs. If have also seen examples of views returning data in CSV formats.
If you are returning HTML in your view, this is where the model data and view markup are married together. Views are meant to be simple and you should not be executing business logic here. That doesn’t mean you cannot have any logic here. So I normally follow this simple rule when creating my views. If the logic pertains to how content is displayed then it’s typically fine.
Separations of Concern in MVC
So I briefly talked about separation of concerns with the MVC pattern. But never answered the question why you might want to separate these components. Below are a few reasons why you would want follow the MVC pattern.
Frontend Designers Can Focus On The View
Frontend Designers typically understand technologies like HTML, CSS, and JavaScript. With this kind of separation, they will be able to focus on the design of the views without having to deal with how the data is retrieved from a database.
Backend Developers Can Focus On The Controller and Model Data
And on the flip side, if you’re a backend coder and you know how to deal with data, getting the data, storing it in a database and so on, then you can deal with the model and controller. A backend developer may be more familiar with technologies like C# or PHP and writing business logic.
Testing
This concept also makes testing easier. If I wanted to test the controller, then I would just need write tests against the controller.
If you have ever used ASP.NET Web Forms before, it had this concept of a code-behind page, and that code-behind page was linked to the HTML code, and that made a very tight coupling. This made it almost impossible to test the logic in this code behind page. And even worse, most developers would store all their business logic here making it impossible to test.
Conclusion
This is a very high-level view of the MVC pattern, and in a future post I will show you how to create the starter code for an ASP.NET MVC project using the MVC framework.
0 Comments